mirror of
https://github.com/ansible-collections/community.general.git
synced 2024-09-14 20:13:21 +02:00
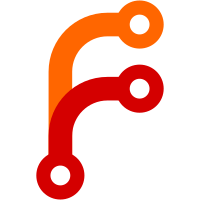
Run setfacl/chown/chmod on each temp dir and file. This fixes temp file permissions handling on platforms such as FreeBSD which always return success when using find -exec. This is done by eliminating the use of find when setting up temp files and directories. Additionally, tests that now pass on FreeBSD have been enabled for CI.
77 lines
2.6 KiB
Python
77 lines
2.6 KiB
Python
# (c) 2015, Brian Coca <briancoca+dev@gmail.com>
|
|
#
|
|
# This file is part of Ansible
|
|
#
|
|
# Ansible is free software: you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation, either version 3 of the License, or
|
|
# (at your option) any later version.
|
|
#
|
|
# Ansible is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
|
|
# Make coding more python3-ish
|
|
from __future__ import (absolute_import, division, print_function)
|
|
__metaclass__ = type
|
|
|
|
import os
|
|
|
|
from ansible.plugins.action import ActionBase
|
|
from ansible.utils.boolean import boolean
|
|
from ansible.errors import AnsibleError
|
|
from ansible.utils.unicode import to_str
|
|
|
|
|
|
class ActionModule(ActionBase):
|
|
|
|
def run(self, tmp=None, task_vars=None):
|
|
if task_vars is None:
|
|
task_vars = dict()
|
|
|
|
result = super(ActionModule, self).run(tmp, task_vars)
|
|
|
|
src = self._task.args.get('src', None)
|
|
remote_src = boolean(self._task.args.get('remote_src', 'no'))
|
|
remote_user = task_vars.get('ansible_ssh_user') or self._play_context.remote_user
|
|
|
|
if src is None:
|
|
result['failed'] = True
|
|
result['msg'] = "src is required"
|
|
return result
|
|
elif remote_src:
|
|
# everything is remote, so we just execute the module
|
|
# without changing any of the module arguments
|
|
result.update(self._execute_module(task_vars=task_vars))
|
|
return result
|
|
|
|
try:
|
|
src = self._find_needle('files', src)
|
|
except AnsibleError as e:
|
|
result['failed'] = True
|
|
result['msg'] = to_str(e)
|
|
return result
|
|
|
|
# create the remote tmp dir if needed, and put the source file there
|
|
if tmp is None or "-tmp-" not in tmp:
|
|
tmp = self._make_tmp_path(remote_user)
|
|
self._cleanup_remote_tmp = True
|
|
|
|
tmp_src = self._connection._shell.join_path(tmp, os.path.basename(src))
|
|
self._transfer_file(src, tmp_src)
|
|
|
|
self._fixup_perms((tmp, tmp_src), remote_user)
|
|
|
|
new_module_args = self._task.args.copy()
|
|
new_module_args.update(
|
|
dict(
|
|
src=tmp_src,
|
|
)
|
|
)
|
|
|
|
result.update(self._execute_module('patch', module_args=new_module_args, task_vars=task_vars))
|
|
self._remove_tmp_path(tmp)
|
|
return result
|