mirror of
https://github.com/ansible-collections/community.general.git
synced 2024-09-14 20:13:21 +02:00
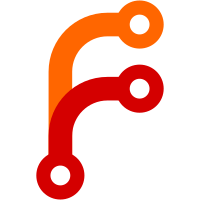
* lxd dynamic inventory and test data * added ``merge_profile`` parameter to merge configurations from the play to an existing profile * cosmetic changes * added ``merge_profile`` parameter to merge configurations from the play to an existing profile * cosmetic changes * fix pylint errors * fix flake8 warnings * fix pep8 errors without "line to long" * fix ansible tests * fix typo * fix version added * fix lost of suggestions from felixfontein * fix filter fix ansible test errors * delete test config * delete 'notes:' and copy content to description * move testdata load testdata by path from config * updated documentation * fix test data and remove inventory branch * fix spellings and rename lxd to community.general.lxd * fix documentation * remove selftest * strip example data * add unit test * switch to ansible.module_utils.common.dict_transformations * documentation cleanup * move lxd_inventory.atd from files to fixtures * update documentation move lxd_inventory.atd * rename self.groups to self dispose remove dumpdata * cleanup * fix unittests comment out dump_data, it breaks the unit tests * fix pep8 * Apply suggestions from code review * Update plugins/inventory/lxd.py * add test if no groupby is selected * rename disposed to groupby remove unused constant other suggested cleanups * Use bundled ipaddress instead of own code. * Update plugins/inventory/lxd.py * Exceptions should not be eaten. * Improve error handling for network range/address parsing. * Fix typo. * Make network range valid. * Do not error when groupby is not a dict. Co-authored-by: Frank Dornheim <“dornheim@posteo.de@users.noreply.github.com”> Co-authored-by: Felix Fontein <felix@fontein.de>
100 lines
3.9 KiB
Python
100 lines
3.9 KiB
Python
# -*- coding: utf-8 -*-
|
|
# Copyright: (c) 2021, Frank Dornheim <dornheim@posteo.de>
|
|
# GNU General Public License v3.0+ (see COPYING or https://www.gnu.org/licenses/gpl-3.0.txt)
|
|
|
|
|
|
from __future__ import (absolute_import, division, print_function)
|
|
__metaclass__ = type
|
|
|
|
import pytest
|
|
import os
|
|
|
|
from ansible.errors import AnsibleError
|
|
from ansible.inventory.data import InventoryData
|
|
from ansible_collections.community.general.plugins.inventory.lxd import InventoryModule
|
|
|
|
|
|
HOST_COMPARATIVE_DATA = {
|
|
'ansible_connection': 'ssh', 'ansible_host': '10.98.143.199', 'ansible_lxd_os': 'ubuntu', 'ansible_lxd_release': 'focal',
|
|
'ansible_lxd_profile': ['default'], 'ansible_lxd_state': 'running', 'ansible_lxd_location': 'Berlin',
|
|
'ansible_lxd_vlan_ids': {'my-macvlan': 666}, 'inventory_hostname': 'vlantest', 'inventory_hostname_short': 'vlantest'}
|
|
GROUP_COMPARATIVE_DATA = {
|
|
'all': [], 'ungrouped': [], 'testpattern': ['vlantest'], 'vlan666': ['vlantest'], 'locationBerlin': ['vlantest'],
|
|
'osUbuntu': ['vlantest'], 'releaseFocal': ['vlantest'], 'releaseBionic': [], 'profileDefault': ['vlantest'],
|
|
'profileX11': [], 'netRangeIPv4': ['vlantest'], 'netRangeIPv6': ['vlantest']}
|
|
GROUP_Config = {
|
|
'testpattern': {'type': 'pattern', 'attribute': 'test'},
|
|
'vlan666': {'type': 'vlanid', 'attribute': 666},
|
|
'locationBerlin': {'type': 'location', 'attribute': 'Berlin'},
|
|
'osUbuntu': {'type': 'os', 'attribute': 'ubuntu'},
|
|
'releaseFocal': {'type': 'release', 'attribute': 'focal'},
|
|
'releaseBionic': {'type': 'release', 'attribute': 'bionic'},
|
|
'profileDefault': {'type': 'profile', 'attribute': 'default'},
|
|
'profileX11': {'type': 'profile', 'attribute': 'x11'},
|
|
'netRangeIPv4': {'type': 'network_range', 'attribute': '10.98.143.0/24'},
|
|
'netRangeIPv6': {'type': 'network_range', 'attribute': 'fd42:bd00:7b11:2167:216:3eff::/96'}}
|
|
|
|
|
|
@pytest.fixture
|
|
def inventory():
|
|
inv = InventoryModule()
|
|
inv.inventory = InventoryData()
|
|
|
|
# Test Values
|
|
inv.data = inv.load_json_data('tests/unit/plugins/inventory/fixtures/lxd_inventory.atd') # Load Test Data
|
|
inv.groupby = GROUP_Config
|
|
inv.prefered_container_network_interface = 'eth'
|
|
inv.prefered_container_network_family = 'inet'
|
|
inv.filter = 'running'
|
|
inv.dump_data = False
|
|
|
|
return inv
|
|
|
|
|
|
def test_verify_file_bad_config(inventory):
|
|
assert inventory.verify_file('foobar.lxd.yml') is False
|
|
|
|
|
|
def test_build_inventory_hosts(inventory):
|
|
"""Load example data and start the inventoryto test the host generation.
|
|
|
|
After the inventory plugin has run with the test data, the result of the host is checked."""
|
|
inventory._populate()
|
|
generated_data = inventory.inventory.get_host('vlantest').get_vars()
|
|
|
|
eq = True
|
|
for key, value in HOST_COMPARATIVE_DATA.items():
|
|
if generated_data[key] != value:
|
|
eq = False
|
|
assert eq
|
|
|
|
|
|
def test_build_inventory_groups(inventory):
|
|
"""Load example data and start the inventory to test the group generation.
|
|
|
|
After the inventory plugin has run with the test data, the result of the host is checked."""
|
|
inventory._populate()
|
|
generated_data = inventory.inventory.get_groups_dict()
|
|
|
|
eq = True
|
|
for key, value in GROUP_COMPARATIVE_DATA.items():
|
|
if generated_data[key] != value:
|
|
eq = False
|
|
assert eq
|
|
|
|
|
|
def test_build_inventory_groups_with_no_groupselection(inventory):
|
|
"""Load example data and start the inventory to test the group generation with groupby is none.
|
|
|
|
After the inventory plugin has run with the test data, the result of the host is checked."""
|
|
inventory.groupby = None
|
|
inventory._populate()
|
|
generated_data = inventory.inventory.get_groups_dict()
|
|
group_comparative_data = {'all': [], 'ungrouped': []}
|
|
|
|
eq = True
|
|
print("data: {0}".format(generated_data))
|
|
for key, value in group_comparative_data.items():
|
|
if generated_data[key] != value:
|
|
eq = False
|
|
assert eq
|