mirror of
https://github.com/ansible-collections/community.general.git
synced 2024-09-14 20:13:21 +02:00
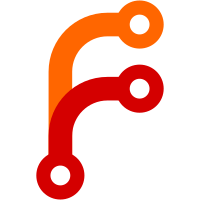
* Using docstrings conflicts with the standard use of docstrings * PYTHON_OPTIMIZE=2 will omit docstrings. Using docstrings makes future changes to the plugin and module code subject to the requirement that we ensure it won't be run with optimization.
50 lines
1.5 KiB
Python
50 lines
1.5 KiB
Python
# (c) 2017 Ansible Project
|
|
# GNU General Public License v3.0+ (see COPYING or https://www.gnu.org/licenses/gpl-3.0.txt)
|
|
|
|
# Make coding more python3-ish
|
|
from __future__ import (absolute_import, division, print_function)
|
|
__metaclass__ = type
|
|
|
|
DOCUMENTATION = '''
|
|
callback: timer
|
|
callback_type: aggregate
|
|
requirements:
|
|
- whitelist in configuration
|
|
short_description: Adds time to play stats
|
|
version_added: "2.0"
|
|
description:
|
|
- This callback just adds total play duration to the play stats.
|
|
'''
|
|
|
|
from datetime import datetime
|
|
|
|
from ansible.plugins.callback import CallbackBase
|
|
|
|
|
|
class CallbackModule(CallbackBase):
|
|
"""
|
|
This callback module tells you how long your plays ran for.
|
|
"""
|
|
CALLBACK_VERSION = 2.0
|
|
CALLBACK_TYPE = 'aggregate'
|
|
CALLBACK_NAME = 'timer'
|
|
CALLBACK_NEEDS_WHITELIST = True
|
|
|
|
def __init__(self):
|
|
|
|
super(CallbackModule, self).__init__()
|
|
|
|
self.start_time = datetime.utcnow()
|
|
|
|
def days_hours_minutes_seconds(self, runtime):
|
|
minutes = (runtime.seconds // 60) % 60
|
|
r_seconds = runtime.seconds % 60
|
|
return runtime.days, runtime.seconds // 3600, minutes, r_seconds
|
|
|
|
def playbook_on_stats(self, stats):
|
|
self.v2_playbook_on_stats(stats)
|
|
|
|
def v2_playbook_on_stats(self, stats):
|
|
end_time = datetime.utcnow()
|
|
runtime = end_time - self.start_time
|
|
self._display.display("Playbook run took %s days, %s hours, %s minutes, %s seconds" % (self.days_hours_minutes_seconds(runtime)))
|