mirror of
https://github.com/ansible-collections/community.general.git
synced 2024-09-14 20:13:21 +02:00
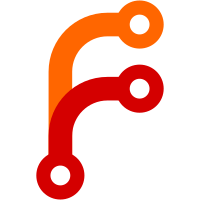
* opennebula: Add template manipulation helpers * one_vm: Use 'updateconf' API call to modify running VMs * one_vm: Emulate 'updateconf' API call for newly created VMs * opennebula/one_vm: Satisfy linter checks * opennebula/one_vm: Apply suggestions from code review Co-authored-by: Alexei Znamensky <103110+russoz@users.noreply.github.com> * opennebula/one_vm: Drop 'extend' function, use 'dict_merge' instead * Add changelog fragment * one_vm: Refactor 'parse_updateconf' function * opennebula/one_vm: Apply suggestions from code review Co-authored-by: Felix Fontein <felix@fontein.de> * one_vm: Allow for using updateconf in all scenarios --------- Co-authored-by: Alexei Znamensky <103110+russoz@users.noreply.github.com> Co-authored-by: Felix Fontein <felix@fontein.de>
62 lines
1.4 KiB
Python
62 lines
1.4 KiB
Python
# -*- coding: utf-8 -*-
|
|
# Copyright (c) 2023, Michal Opala <mopala@opennebula.io>
|
|
# GNU General Public License v3.0+ (see LICENSES/GPL-3.0-or-later.txt or https://www.gnu.org/licenses/gpl-3.0.txt)
|
|
# SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
from __future__ import (absolute_import, division, print_function)
|
|
__metaclass__ = type
|
|
|
|
import os
|
|
|
|
import pytest
|
|
|
|
from ansible_collections.community.general.plugins.modules.one_vm import parse_updateconf
|
|
|
|
|
|
PARSE_UPDATECONF_VALID = [
|
|
(
|
|
{
|
|
"CPU": 1,
|
|
"OS": {"ARCH": 2},
|
|
},
|
|
{
|
|
"OS": {"ARCH": 2},
|
|
}
|
|
),
|
|
(
|
|
{
|
|
"OS": {"ARCH": 1, "ASD": 2}, # "ASD" is an invalid attribute, we ignore it
|
|
},
|
|
{
|
|
"OS": {"ARCH": 1},
|
|
}
|
|
),
|
|
(
|
|
{
|
|
"OS": {"ASD": 1}, # "ASD" is an invalid attribute, we ignore it
|
|
},
|
|
{
|
|
}
|
|
),
|
|
(
|
|
{
|
|
"MEMORY": 1,
|
|
"CONTEXT": {
|
|
"PASSWORD": 2,
|
|
"SSH_PUBLIC_KEY": 3,
|
|
},
|
|
},
|
|
{
|
|
"CONTEXT": {
|
|
"PASSWORD": 2,
|
|
"SSH_PUBLIC_KEY": 3,
|
|
},
|
|
}
|
|
),
|
|
]
|
|
|
|
|
|
@pytest.mark.parametrize('vm_template,expected_result', PARSE_UPDATECONF_VALID)
|
|
def test_parse_updateconf(vm_template, expected_result):
|
|
result = parse_updateconf(vm_template)
|
|
assert result == expected_result, repr(result)
|