mirror of
https://github.com/ansible-collections/community.general.git
synced 2024-09-14 20:13:21 +02:00
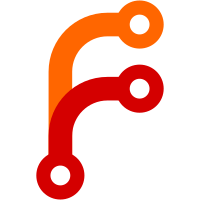
* Add `ignore_spaces` option to `ini_file` to ignore spacing changes Add a new `ignore_spaces` option to the `ini_file` module which, if true, prevents the module from changing a line in a file if the only thing that would change by doing so is whitespace before or after the `=`. Also add test cases for this new functionality. There were previously no tests for `ini_file` at all, and this doesn't attempt to fix that, but it does add tests to ensure that the new behavior implemented here as well as the old behavior in the affected code are correct. Fixes #7202. * Add changelog fragment * pep8 / pylint * remove unused import * fix typo in comment in integration test file * Add symlink tests to main.yml It appears that #6546 added symlink tests but neglected to add them to main.yml so they weren't being executed. * ini_file symlink tests; create output files in correct location * Add integration tests for ini_file ignore_spaces * PR feedback
51 lines
1.6 KiB
Python
51 lines
1.6 KiB
Python
# Copyright (c) 2023 Ansible Project
|
|
# GNU General Public License v3.0+ (see LICENSES/GPL-3.0-or-later.txt or
|
|
# https://www.gnu.org/licenses/gpl-3.0.txt)
|
|
# SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
from __future__ import (absolute_import, division, print_function)
|
|
__metaclass__ = type
|
|
|
|
from ansible_collections.community.general.plugins.modules import ini_file
|
|
|
|
|
|
def do_test(option, ignore_spaces, newline, before, expected_after,
|
|
expected_changed, expected_msg):
|
|
section_lines = [before]
|
|
changed_lines = [0]
|
|
changed, msg = ini_file.update_section_line(
|
|
option, None, section_lines, 0, changed_lines, ignore_spaces,
|
|
newline, None)
|
|
assert section_lines[0] == expected_after
|
|
assert changed == expected_changed
|
|
assert changed_lines[0] == 1
|
|
assert msg == expected_msg
|
|
|
|
|
|
def test_ignore_spaces_comment():
|
|
oldline = ';foobar=baz'
|
|
newline = 'foobar = baz'
|
|
do_test('foobar', True, newline, oldline, newline, True, 'option changed')
|
|
|
|
|
|
def test_ignore_spaces_changed():
|
|
oldline = 'foobar=baz'
|
|
newline = 'foobar = freeble'
|
|
do_test('foobar', True, newline, oldline, newline, True, 'option changed')
|
|
|
|
|
|
def test_ignore_spaces_unchanged():
|
|
oldline = 'foobar=baz'
|
|
newline = 'foobar = baz'
|
|
do_test('foobar', True, newline, oldline, oldline, False, None)
|
|
|
|
|
|
def test_no_ignore_spaces_changed():
|
|
oldline = 'foobar=baz'
|
|
newline = 'foobar = baz'
|
|
do_test('foobar', False, newline, oldline, newline, True, 'option changed')
|
|
|
|
|
|
def test_no_ignore_spaces_unchanged():
|
|
newline = 'foobar=baz'
|
|
do_test('foobar', False, newline, newline, newline, False, None)
|