mirror of
https://github.com/ansible-collections/community.general.git
synced 2024-09-14 20:13:21 +02:00
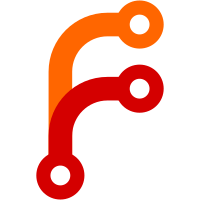
The goal of breaking apart the base_parser() function is to get rid of a bunch of conditionals and parameters in the code and, instead, make code look like simple composition. When splitting, a choice had to be made as to whether this would operate by side effect (modifying a passed in parser) or side effect-free (returning a new parser everytime). Making a version that's side-effect-free appears to be fighting with the optparse API (it wants to work by creating a parser object, configuring the object, and then parsing the arguments with it) so instead, make it clear that our helper functions are modifying the passed in parser by (1) not returning the parser and (2) changing the function names to be more clear that it is operating by side-effect. Also move all of the generic optparse code, along with the argument context classes, into a new subdirectory.
91 lines
3.5 KiB
Python
91 lines
3.5 KiB
Python
# -*- coding: utf-8 -*-
|
|
# Copyright: (c) 2018, Toshio Kuratomi <tkuratomi@ansible.com>
|
|
# GNU General Public License v3.0+ (see COPYING or https://www.gnu.org/licenses/gpl-3.0.txt)
|
|
|
|
# Make coding more python3-ish
|
|
from __future__ import (absolute_import, division)
|
|
__metaclass__ = type
|
|
|
|
try:
|
|
import argparse
|
|
except ImportError:
|
|
argparse = None
|
|
|
|
import optparse
|
|
|
|
import pytest
|
|
|
|
from ansible.arguments import context_objects as co
|
|
from ansible.module_utils.common.collections import ImmutableDict
|
|
|
|
|
|
MAKE_IMMUTABLE_DATA = ((u'くらとみ', u'くらとみ'),
|
|
(42, 42),
|
|
({u'café': u'くらとみ'}, ImmutableDict({u'café': u'くらとみ'})),
|
|
([1, u'café', u'くらとみ'], (1, u'café', u'くらとみ')),
|
|
(set((1, u'café', u'くらとみ')), frozenset((1, u'café', u'くらとみ'))),
|
|
({u'café': [1, set(u'ñ')]},
|
|
ImmutableDict({u'café': (1, frozenset(u'ñ'))})),
|
|
([set((1, 2)), {u'くらとみ': 3}],
|
|
(frozenset((1, 2)), ImmutableDict({u'くらとみ': 3}))),
|
|
)
|
|
|
|
|
|
@pytest.mark.parametrize('data, expected', MAKE_IMMUTABLE_DATA)
|
|
def test_make_immutable(data, expected):
|
|
assert co._make_immutable(data) == expected
|
|
|
|
|
|
def test_cliargs_from_dict():
|
|
old_dict = {'tags': [u'production', u'webservers'],
|
|
'check_mode': True,
|
|
'start_at_task': u'Start with くらとみ'}
|
|
expected = frozenset((('tags', (u'production', u'webservers')),
|
|
('check_mode', True),
|
|
('start_at_task', u'Start with くらとみ')))
|
|
|
|
assert frozenset(co.CLIArgs(old_dict).items()) == expected
|
|
|
|
|
|
def test_cliargs():
|
|
class FakeOptions:
|
|
pass
|
|
options = FakeOptions()
|
|
options.tags = [u'production', u'webservers']
|
|
options.check_mode = True
|
|
options.start_at_task = u'Start with くらとみ'
|
|
|
|
expected = frozenset((('tags', (u'production', u'webservers')),
|
|
('check_mode', True),
|
|
('start_at_task', u'Start with くらとみ')))
|
|
|
|
assert frozenset(co.CLIArgs.from_options(options).items()) == expected
|
|
|
|
|
|
@pytest.mark.skipIf(argparse is None)
|
|
def test_cliargs_argparse():
|
|
parser = argparse.ArgumentParser(description='Process some integers.')
|
|
parser.add_argument('integers', metavar='N', type=int, nargs='+',
|
|
help='an integer for the accumulator')
|
|
parser.add_argument('--sum', dest='accumulate', action='store_const',
|
|
const=sum, default=max,
|
|
help='sum the integers (default: find the max)')
|
|
args = parser.parse_args([u'--sum', u'1', u'2'])
|
|
|
|
expected = frozenset((('accumulate', sum), ('integers', (1, 2))))
|
|
|
|
assert frozenset(co.CLIArgs.from_options(args).items()) == expected
|
|
|
|
|
|
# Can get rid of this test when we port ansible.cli from optparse to argparse
|
|
def test_cliargs_optparse():
|
|
parser = optparse.OptionParser(description='Process some integers.')
|
|
parser.add_option('--sum', dest='accumulate', action='store_const',
|
|
const=sum, default=max,
|
|
help='sum the integers (default: find the max)')
|
|
opts, args = parser.parse_args([u'--sum', u'1', u'2'])
|
|
opts.integers = args
|
|
|
|
expected = frozenset((('accumulate', sum), ('integers', (u'1', u'2'))))
|
|
|
|
assert frozenset(co.CLIArgs.from_options(opts).items()) == expected
|