mirror of
https://github.com/ansible-collections/community.general.git
synced 2024-09-14 20:13:21 +02:00
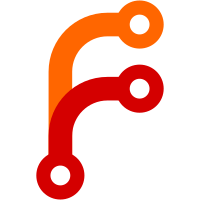
* Remote management modules for OCAPI-based devices. Open Composable API (OCAPI) is a REST-based API designed for data center composability. For more information, see https://www.opencompute.org/documents/open-composable-api-for-ocp-2019-06-24-pdf This PR introduces ocapi_command and ocapi_info modules. These are based on the existing redfish_command and redfish_info modules and follow similar patterns. This initial implementation includes support for the folowing operations: - Indicator LED toggling - Power state toggling - Enclosure reset (reboot) - Firmware upload - Firmware update - Firmware activate - Job deletion - Job status These modules have been tested against Western Digital OpenFlex(tm) Data24 storage enclosures. API reference is at https://documents.westerndigital.com/content/dam/doc-library/en_us/assets/public/western-digital/product/platforms/openflex/reference-architecture-open-composable-api.pdf * Fix licensing issue for ocapi_utils.py * PR Feedback * Apply suggestions from code review Co-authored-by: Felix Fontein <felix@fontein.de> * Update plugins/module_utils/ocapi_utils.py Co-authored-by: Felix Fontein <felix@fontein.de> * Update plugins/modules/ocapi_info.py Co-authored-by: Felix Fontein <felix@fontein.de> * Apply suggestions from code review Co-authored-by: Felix Fontein <felix@fontein.de> * PR Feedback Use six module for urlparse * Apply suggestions from code review Documentation fixes. Co-authored-by: Felix Fontein <felix@fontein.de> * Fix sanity test line too long error. Co-authored-by: Felix Fontein <felix@fontein.de>
54 lines
2.2 KiB
Python
54 lines
2.2 KiB
Python
# -*- coding: utf-8 -*-
|
|
# Copyright (c) Ansible project
|
|
# GNU General Public License v3.0+ (see LICENSES/GPL-3.0-or-later.txt or https://www.gnu.org/licenses/gpl-3.0.txt)
|
|
# SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
from __future__ import (absolute_import, division, print_function)
|
|
__metaclass__ = type
|
|
|
|
import os
|
|
import re
|
|
import shutil
|
|
import tempfile
|
|
|
|
from ansible_collections.community.general.tests.unit.compat import unittest
|
|
from ansible_collections.community.general.plugins.module_utils.ocapi_utils import OcapiUtils
|
|
|
|
|
|
class TestOcapiUtils(unittest.TestCase):
|
|
def setUp(self):
|
|
self.tempdir = tempfile.mkdtemp()
|
|
self.utils = OcapiUtils(creds={"user": "a_user", "pswd": "a_password"},
|
|
base_uri="fakeUri",
|
|
proxy_slot_number=None,
|
|
timeout=30,
|
|
module=None)
|
|
|
|
def tearDown(self):
|
|
shutil.rmtree(self.tempdir)
|
|
|
|
def test_prepare_multipart_firmware_upload(self):
|
|
# Generate a binary file and save it
|
|
filename = "fake_firmware.bin"
|
|
filepath = os.path.join(self.tempdir, filename)
|
|
file_contents = b'\x00\x01\x02\x03\x04'
|
|
with open(filepath, 'wb+') as f:
|
|
f.write(file_contents)
|
|
|
|
# Call prepare_mutipart_firmware_upload
|
|
content_type, b_form_data = self.utils.prepare_multipart_firmware_upload(filepath)
|
|
|
|
# Check the returned content-type
|
|
content_type_pattern = r"multipart/form-data; boundary=(.*)"
|
|
m = re.match(content_type_pattern, content_type)
|
|
self.assertIsNotNone(m)
|
|
|
|
# Check the returned binary data
|
|
boundary = m.group(1)
|
|
expected_content_text = '--%s\r\n' % boundary
|
|
expected_content_text += 'Content-Disposition: form-data; name="FirmwareFile"; filename="%s"\r\n' % filename
|
|
expected_content_text += 'Content-Type: application/octet-stream\r\n\r\n'
|
|
expected_content_bytes = bytearray(expected_content_text, 'utf-8')
|
|
expected_content_bytes += file_contents
|
|
expected_content_bytes += bytearray('\r\n--%s--' % boundary, 'utf-8')
|
|
self.assertEqual(expected_content_bytes, b_form_data)
|