mirror of
https://github.com/ansible-collections/community.general.git
synced 2024-09-14 20:13:21 +02:00
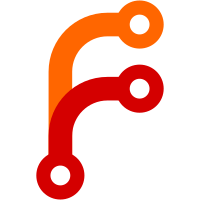
This flag will show playbook output from non-failing commands. -v is also added to /usr/bin/ansible, but not yet used. I also gutted some internals code dealing with 'invocations' which allowed the callback to know what module invoked it. This is not something 0.5 does or needed, so callbacks have been simplified.
134 lines
4.5 KiB
Python
Executable file
134 lines
4.5 KiB
Python
Executable file
#!/usr/bin/env python
|
|
|
|
# (c) 2012, Michael DeHaan <michael.dehaan@gmail.com>
|
|
#
|
|
# This file is part of Ansible
|
|
#
|
|
# Ansible is free software: you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation, either version 3 of the License, or
|
|
# (at your option) any later version.
|
|
#
|
|
# Ansible is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with Ansible. If not, see <http://www.gnu.org/licenses/>.
|
|
|
|
########################################################
|
|
|
|
import sys
|
|
import getpass
|
|
|
|
from ansible.runner import Runner
|
|
import ansible.constants as C
|
|
from ansible import utils
|
|
from ansible import errors
|
|
from ansible import callbacks
|
|
from ansible import inventory
|
|
|
|
########################################################
|
|
|
|
class Cli(object):
|
|
''' code behind bin/ansible '''
|
|
|
|
# ----------------------------------------------
|
|
|
|
def __init__(self):
|
|
self.stats = callbacks.AggregateStats()
|
|
self.callbacks = callbacks.CliRunnerCallbacks()
|
|
|
|
# ----------------------------------------------
|
|
|
|
def parse(self):
|
|
''' create an options parser for bin/ansible '''
|
|
|
|
parser = utils.base_parser(constants=C, runas_opts=True, async_opts=True,
|
|
output_opts=True, connect_opts=True, usage='%prog <host-pattern> [options]')
|
|
parser.add_option('-a', '--args', dest='module_args',
|
|
help="module arguments", default=C.DEFAULT_MODULE_ARGS)
|
|
parser.add_option('-m', '--module-name', dest='module_name',
|
|
help="module name to execute (default=%s)" % C.DEFAULT_MODULE_NAME,
|
|
default=C.DEFAULT_MODULE_NAME)
|
|
options, args = parser.parse_args()
|
|
self.callbacks.options = options
|
|
|
|
if len(args) == 0 or len(args) > 1:
|
|
parser.print_help()
|
|
sys.exit(1)
|
|
return (options, args)
|
|
|
|
# ----------------------------------------------
|
|
|
|
def run(self, options, args):
|
|
''' use Runner lib to do SSH things '''
|
|
|
|
pattern = args[0]
|
|
|
|
inventory_manager = inventory.Inventory(options.inventory)
|
|
hosts = inventory_manager.list_hosts(pattern)
|
|
if len(hosts) == 0:
|
|
print >>sys.stderr, "No hosts matched"
|
|
sys.exit(1)
|
|
|
|
sshpass = None
|
|
sudopass = None
|
|
if options.ask_pass:
|
|
sshpass = getpass.getpass(prompt="SSH password: ")
|
|
if options.ask_sudo_pass:
|
|
sudopass = getpass.getpass(prompt="sudo password: ")
|
|
options.sudo = True
|
|
if options.sudo_user:
|
|
options.sudo = True
|
|
options.sudo_user = options.sudo_user or C.DEFAULT_SUDO_USER
|
|
if options.tree:
|
|
utils.prepare_writeable_dir(options.tree)
|
|
|
|
runner = Runner(
|
|
module_name=options.module_name, module_path=options.module_path,
|
|
module_args=options.module_args,
|
|
remote_user=options.remote_user, remote_pass=sshpass,
|
|
inventory=inventory_manager, timeout=options.timeout,
|
|
private_key_file=options.private_key_file,
|
|
forks=options.forks,
|
|
pattern=pattern,
|
|
callbacks=self.callbacks, sudo=options.sudo,
|
|
sudo_pass=sudopass,sudo_user=options.sudo_user,
|
|
transport=options.connection, verbose=options.verbose
|
|
)
|
|
|
|
if options.seconds:
|
|
print "background launch...\n\n"
|
|
results, poller = runner.run_async(options.seconds)
|
|
results = self.poll_while_needed(poller, options)
|
|
else:
|
|
results = runner.run()
|
|
|
|
return (runner, results)
|
|
|
|
# ----------------------------------------------
|
|
|
|
def poll_while_needed(self, poller, options):
|
|
''' summarize results from Runner '''
|
|
|
|
# BACKGROUND POLL LOGIC when -B and -P are specified
|
|
if options.seconds and options.poll_interval > 0:
|
|
poller.wait(options.seconds, options.poll_interval)
|
|
|
|
return poller.results
|
|
|
|
|
|
########################################################
|
|
|
|
if __name__ == '__main__':
|
|
cli = Cli()
|
|
(options, args) = cli.parse()
|
|
try:
|
|
(runner, results) = cli.run(options, args)
|
|
except errors.AnsibleError, e:
|
|
# Generic handler for ansible specific errors
|
|
print "ERROR: %s" % str(e)
|
|
sys.exit(1)
|
|
|